Checkbox
A checkbox allows the user to check or uncheck an option.
Anatomy
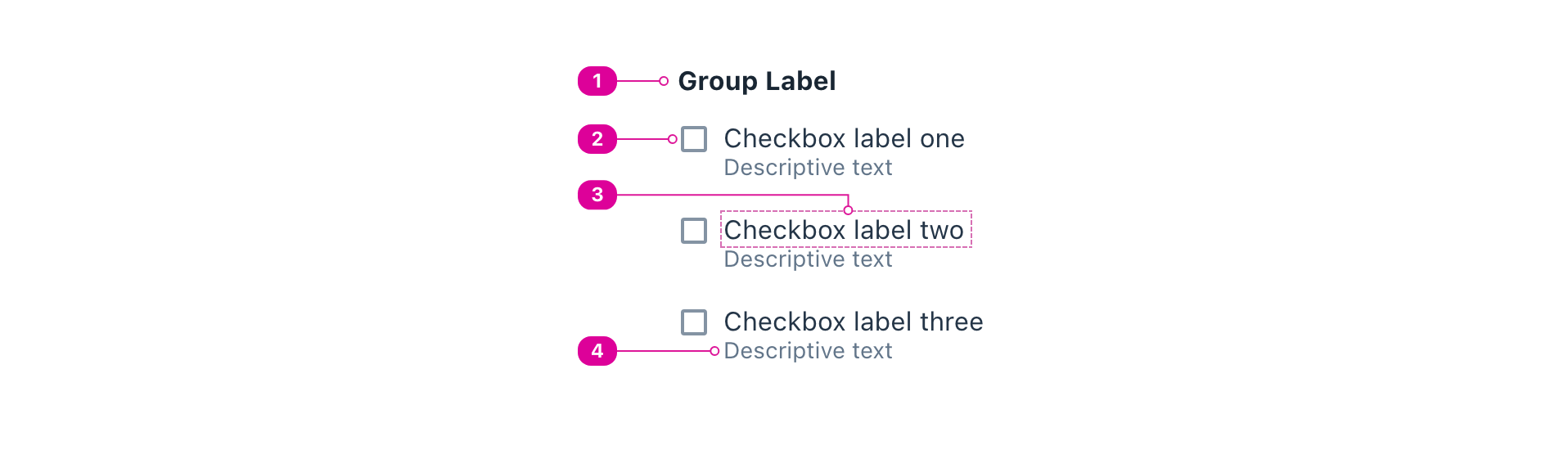
- Group Label - text that conveys how the checkboxes are related and prompts the user to check one or more checkbox.
- Control - the input control that conveys the appropriate state of the checkbox to the user. By default it is unselected. Clicking a checkbox will check or uncheck it.
- Checkbox Label - a short name that lets the user know what the checkbox is checking. Clicking the label should toggle the checkbox.
- Description (optional) - additional text that can be used to add clarity or context to the checkbox label. Clicking the description should do nothing.
Usage
- A single checkbox has two mutually exclusive states: checked or unchecked.
- Space toggles the state of the checkbox.
- Use a group of checkboxes to allow a user to check zero, one or multiple options from a list, and display all available options.
- Checkboxes may allow users to sub-select options from a nested list (see tri-state checkboxes).
- Checkboxes work independently of other checkboxes, unless they are controlled by a tri-state checkbox.
- Avoid setting default options, because pre-checking an option makes it more likely that users will not make a conscious choice and submit an option unintentionally.
- Consider the most logical order to list options. Options may be listed alphabetically, by popularity, or some other ordered system that should be clear to the user.
Tri-state Checkbox
A tri-state checkbox can be used to represent and control the state of a group of other checkboxes. This type of checkbox adds an "indeterminate" state that conveys to the user that some—but not all—of the related checkboxes are checked.
- If all options in the group are checked, the tri-state checkbox displays as checked.
- If some of the options in the group are checked, the tri-state checkbox displays as indeterminate (partially checked).
- If none of the options in the group are checked, the tri-state checkbox displays as unchecked.
- If a user checks a tri-state checkbox, then all the options in the group are checked.
- If a user unchecks a tri-state checkbox, then all the options in the group are unchecked.
React API
import { Checkbox, CheckboxGroup } from '@wwnds/react';
Checkbox
A single checkbox component.
<Checkbox description="Additional information about this checkbox." required requiredIndicator > Checkbox </Checkbox>
Name | Description | Type |
---|---|---|
baseName | The base class name according to BEM conventions. | string |
description | An optional description. Use this in place of | ReactNode |
labelClass | A className for the label element, which will be a | string |
descriptionClass | A className for the description <div> . | string |
labelId | An id for the label element. | string |
descriptionId | An id for the description <div> . | string |
errors | A list of error strings. If provided, this will be set as an unordered list in the first child slot. | string[] |
validators | A list of validators. A validator contains a function that tests the value for validity and a corresponding message that conveys why the test failed. | ValidatorEntry[] |
validateOnDOMChange | Indicates that validation should occur when the DOM's Reference: | boolean |
validateOnChange | Indicates that validation should occur when | boolean |
maxLengthRestrictsInput | Indicates that a maxLength value should prevent input beyond the maxLength . | boolean |
onDOMChange | A callback that will be triggered any time the DOM's Reference: | ((e: Event) => void) |
onValidate | A callback that will be triggered any time the input is validated. See
related | ((errors: string[]) => void) |
indeterminate | Mark the checkbox as indeterminate. Has no effect when Reference: | boolean |
requiredIndicator | Indicates that the indicator should be "required" when required=true . | boolean |
optionalIndicator | Indicates that the indicator should be "optional" when required=false . | boolean |
thumbnail | The thumbnail element. | ReactNode |
controlClass | The className for the control that sighted users will see. | string |
inputClass | The className for the Checkbox's <input> element. | string |
thumbnailClass | The className for the Checkbox's thumbnail element. | string |
checkedClass | A className that will be applied to the root of the component when it is checked. | string |
errorsClass | A className for the error list. | string |
errorsId | An id for the error list. | string |
CheckboxGroup
A container for a set of related Checkbox
components and its associated prompt (label
).
<CheckboxGroup label="Select your favorite fruits." name="fruit"> <Checkbox thumbnail="🍎" description="A classic choice">Apple</Checkbox> <Checkbox thumbnail="🍌" description="A taste of the tropics">Banana</Checkbox> <Checkbox thumbnail="🥝" description="A bit more exotic">Kiwi</Checkbox> <Checkbox thumbnail="🍊" description="Tangy and juicy">Orange</Checkbox> </CheckboxGroup>
Name | Description | Type |
---|---|---|
label (required) | Text that conveys how the choices are related and prompts the user to choose one or more choice. | ReactNode |
baseName | The base class name according to BEM conventions. | string |
name | The name that will be assigned to all child <input> elements. | string |
description | An optional description. Use this in place of | ReactNode |
labelClass | A className for the label element, which will be a | string |
descriptionClass | A className for the description <div> . | string |
labelId | An id for the label element. | string |
descriptionId | An id for the description <div> . | string |
required | Indicates whether a selection must be made or not. | boolean |
errors | A list of error strings. If provided, this will be set as an unordered list in the first child slot. | string[] |
requiredIndicator | Indicates that the indicator should be "required" when required=true . | boolean |
optionalIndicator | Indicates that the indicator should be "optional" when required=false . | boolean |
errorsClass | A className for the error list. | string |
errorsId | An id for the error list. | string |
fieldName | The name that will be assigned to the parent <fieldset> . | string |
choiceClass | The class name that will be used on all Choice elements. | string |