Radio Group
A radio group allows the user to select one option from a set of related options.
Anatomy
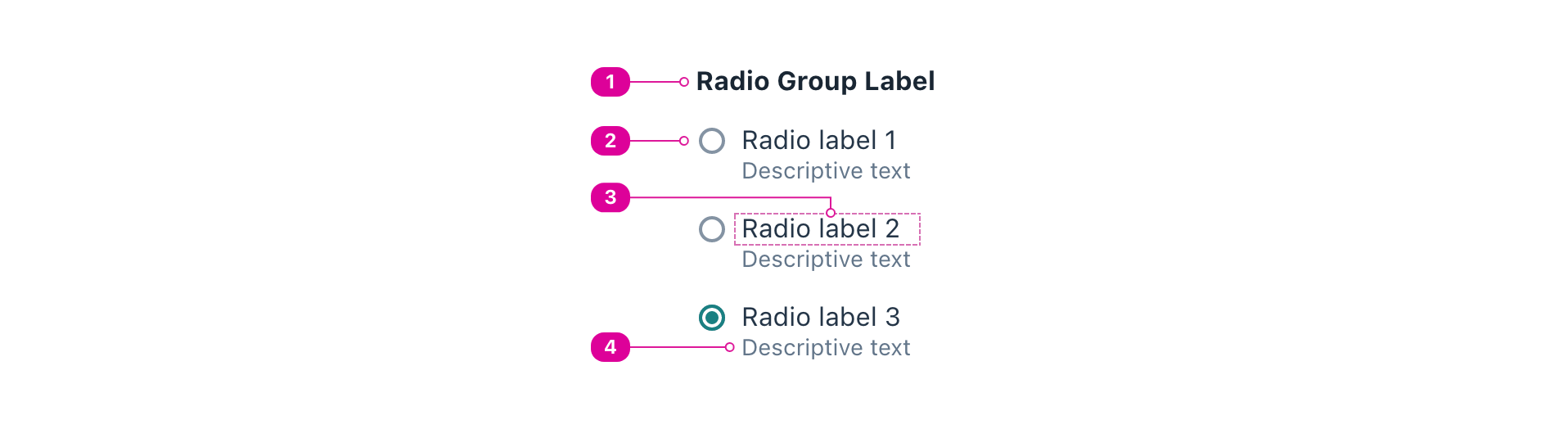
- Group Label - text that conveys how the radio buttons are related and prompts the user to select a single radio button.
- Control - the input control that conveys the current state of the radio button to the user. A selected radio button indicates that it will be the option that is submitted.
- Radio Button Label - a short name that lets the user know what the radio button is selecting. Clicking the label should select the radio button.
- Description (optional) - additional text that can be used to add clarity or context to the radio button label. Clicking the description should do nothing.
Usage
- Use radio buttons to allow a user to select exactly one item from a set of options, and display all available options.
- Radio buttons should have between 2-5 options.
- If you have more than 5 options, use a dropdown or combo box.
- The options in a group of radio buttons must be mutually exclusive. The user can only select 1 Radio.
- Only use radio buttons when the field is required. If all radio buttons are in an unselected state on load, a user must select one of the radio buttons before proceeding.
- Arrow keys move focus within the group, uncheck the previously focused button, and check the newly focused button.
- Space selects the focused radio button if it is not already selected.
React API
import { Radio, RadioGroup } from '@wwnds/react';
RadioGroup
<RadioGroup label="Select your favorite fruit." name="fruit"> <Radio thumbnail="🍎" description="A classic choice">Apple</Radio> <Radio thumbnail="🍌" description="A taste of the tropics">Banana</Radio> <Radio thumbnail="🥝" description="A bit more exotic">Kiwi</Radio> <Radio thumbnail="🍊" description="Tangy and juicy">Orange</Radio> </RadioGroup>
Name | Description | Type |
---|---|---|
label (required) | Text that conveys how the choices are related and prompts the user to choose one or more choice. | ReactNode |
baseName | The base class name according to BEM conventions. | string |
name | The name that will be assigned to all child <input> elements. | string |
description | An optional description. Use this in place of | ReactNode |
labelClass | A className for the label element, which will be a | string |
descriptionClass | A className for the description <div> . | string |
labelId | An id for the label element. | string |
descriptionId | An id for the description <div> . | string |
required | Indicates whether a selection must be made or not. | boolean |
errors | A list of error strings. If provided, this will be set as an unordered list in the first child slot. | string[] |
requiredIndicator | Indicates that the indicator should be "required" when required=true . | boolean |
optionalIndicator | Indicates that the indicator should be "optional" when required=false . | boolean |
errorsClass | A className for the error list. | string |
errorsId | An id for the error list. | string |
fieldName | The name that will be assigned to the parent <fieldset> . | string |
choiceClass | The class name that will be used on all Choice elements. | string |
Radio
Note: there is no scenario where you would use a single Radio
alone.
<Radio>Apple</Radio>
Name | Description | Type |
---|---|---|
baseName | The base class name according to BEM conventions. | string |
description | An optional description. Use this in place of | ReactNode |
labelClass | A className for the label element, which will be a | string |
descriptionClass | A className for the description <div> . | string |
labelId | An id for the label element. | string |
descriptionId | An id for the description <div> . | string |
errors | A list of error strings. If provided, this will be set as an unordered list in the first child slot. | string[] |
validators | A list of validators. A validator contains a function that tests the value for validity and a corresponding message that conveys why the test failed. | ValidatorEntry[] |
validateOnDOMChange | Indicates that validation should occur when the DOM's Reference: | boolean |
validateOnChange | Indicates that validation should occur when | boolean |
maxLengthRestrictsInput | Indicates that a maxLength value should prevent input beyond the maxLength . | boolean |
onDOMChange | A callback that will be triggered any time the DOM's Reference: | ((e: Event) => void) |
onValidate | A callback that will be triggered any time the input is validated. See
related | ((errors: string[]) => void) |
indeterminate | Mark the checkbox as indeterminate. Has no effect when Reference: | boolean |
requiredIndicator | Indicates that the indicator should be "required" when required=true . | boolean |
optionalIndicator | Indicates that the indicator should be "optional" when required=false . | boolean |
thumbnail | The thumbnail element. | ReactNode |
controlClass | The className for the control that sighted users will see. | string |
inputClass | The className for the Checkbox's <input> element. | string |
thumbnailClass | The className for the Checkbox's thumbnail element. | string |
checkedClass | A className that will be applied to the root of the component when it is checked. | string |
errorsClass | A className for the error list. | string |
errorsId | An id for the error list. | string |